Building a turn-based minigame with an 'NPC'
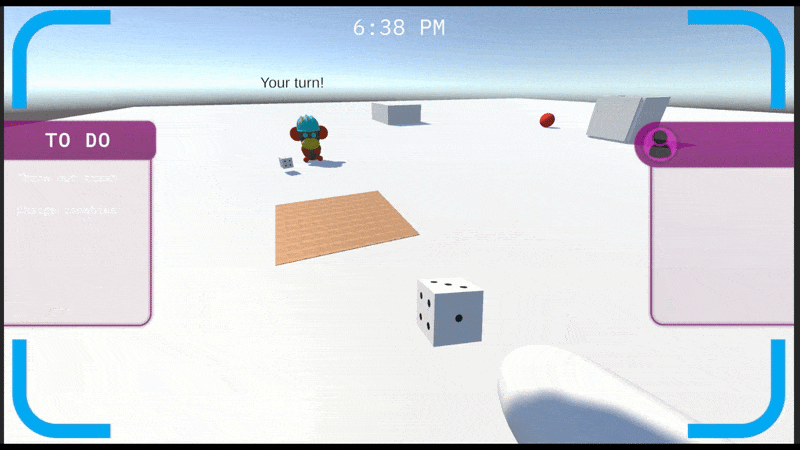
As the production phase continues, we've established a handful of minigames that will form the core of our experience. One of my features is the dice minigame, where players compete against monkey Jim in a fake casino environment. My objective this devlog cycle was to find a way to rig some of Jim's rolls (as all casinos go, of course), and it was a bit more difficult than I had anticipated. This, plus finding the rest of the sound effects for the basketball game, rounded out my last few weeks.
Every version of the dice roll felt off. I tried playing around with Rotate(), DoTween's DoRotate() (where you can feed a certain angular rotation in, with the ability to rotate past 360), and even having the object lerp to a rotation amidst the randomness of the physics-based roll, but nothing worked. I needed to find a 'natural' implementation that retained the physics feel, so that it doesn't interfere with, or require reworking of, the throw system.
So, this might look like just a random dice roll. But, it's been rigged so that Jim is guaranteed to roll either equal to or less than the player, meaning that he is guaranteed to lose. I managed to find an implementation that uses built-in physics and is 100% consistent.
So how does it work? Well, Unity has the ability to run a Physics simulation via script. This means that I could simulate a roll to determine the result. Then, I could use that result to determine a certain amount of rotation to apply to the dice to ensure that it lands on the correct 'up' face. But then, the simulation doesn't have the necessary rotation as it happens.
The final piece of the puzzle is creating a sim recorder. This script can track position and rotation data in a given frame, and save it to be replayed when called. With this, I record the physics simulation, replay it with an altered additional relative rotation from the current to desired face (based on the previously calculated 'up' from the simulation), replay it, and voila! A rigged dice roll.
public void StartRecording(Vector3 force, Vector3 torque) { Physics.simulationMode = SimulationMode.Script; //add forces recordingData.rb.AddForce(force, ForceMode.Impulse); recordingData.rb.AddTorque(torque, ForceMode.Impulse); //needs to simulate one frame first (so force is added), stop recording when object comes to rest do { recordingData.recordedAnimation.Add(new RecordedFrame(transform.position, transform.rotation)); Physics.Simulate(Time.fixedDeltaTime); } while (recordingData.rb.velocity.magnitude > 0.01f && recordingData.rb.angularVelocity.magnitude > 0.01f); //save the new up for the rotation calculation finalUp = GetComponent<dice>().CalculateNewUp(); Physics.simulationMode = SimulationMode.FixedUpdate; }
Here's the function for the physics simulation recording. It's tailored to work for this dice roll, given that it stops recording when the object falls to rest, but it can be modified for other uses as well. The rest of the minigame runs on a lot of coroutines for delays and WaitUntil's to ensure things fire at the right time.
Now all we need to do is juice this up to 100 by giving Jim a suit, poker table, flashy lights and lots of money sound effects, and we'll have our own little casino game!
Oh, and I found sound effects for the basketball game. Whee!
Get My Ordinary AR Life
My Ordinary AR Life
Status | Prototype |
Authors | Braeden, Jonathan To, Arc Zhu, Naitoshadou, St2ele |
Genre | Puzzle |
Tags | Comedy, Puzzle-Platformer |
More posts
- Hudson Steele: Dev Log 3 - Quality Controls18 days ago
- Rose Lazuli - Production Devlog 3: Learning and Improving19 days ago
- Dev Log 3 - HUD Animations and Mini-Game Progress19 days ago
- Dev Log 2 - Virtual Environment Interactions53 days ago
- Devlog 2 - Audio Implementation54 days ago
- Devlog - Designing Minigames and Creating Assets57 days ago
- Rose Lazuli - Production Devlog 258 days ago
- Dev Log 1 - Into Digital Virtual Reality71 days ago
- Devlog 1 - Making a Dialogue System75 days ago